Android Get Download Cache Directory Permission
- Warframe Stuck At Verifying Download Cache
- Clear Download Cache
- Android Request Permission
- Download Cache Cleaner For Pc
- Download Cache Size Chart
- Warframe Optimizing Download Cache
- Android Get Download Cache Directory Permission
- Android Developer Permissions
belongs to Maven artifact com.android.support:support-compat:28.0.0-alpha1
Cache directory is the application specific directory on the file system where the files in this directory get deleted first when the device runs low on storage. In this article, we will create an Android application which allows users to input some content into an EditText widget and store those content in a file located in the temporary. However, CacheManager.getCacheFileBaseDir() always returns null. Do I need to create the cache folder in the android emulator? If yes, how can. Stack Overflow. Log In Sign Up; current community. My cache folder in Android is null. Do I have to create it? Do I need to add any permission in order to use the cache apart from the. Just how to get plain vanilla android on a purchased device. – bgs Jan 6 '11 at 19:20 No such prompt occurs on my Android 7.1.1 device. But adb goes ahead and lists contents of the directory.
How to get my Android device Internal Download Folder path [duplicate]. How to access downloads folder in android? 3 answers It is possible to get the Android device Internal Download Folder path? Android download storage internal. Permission android:name='android.permission.WRITE_EXTERNAL_STORAGE' /> Happy coding. Return the download/cache content directory. Static File: getExternalStorageDirectory() Return the primary shared/external storage directory. Writing to this path requires the Manifest.permission.WRITE_EXTERNAL_STORAGE permission. Get Android and Google Play news by email. Android Saving Files on Internal and External Storage. We can open a File object representing the cache directory in the internal storage by using the getCacheDir() method. Let’s see some code to create cache files. 10 thoughts on “Android Saving Files on Internal and External Storage” john says: April 15, 2015 at 2:56 PM.
public class FileProvider
extends ContentProvider
java.lang.Object | ||
↳ | android.content.ContentProvider | |
↳ | android.support.v4.content.FileProvider |
FileProvider is a special subclass of ContentProvider
that facilitates secure sharing of files associated with an app by creating a content://
Uri
for a file instead of a file:///
Uri
.
A content URI allows you to grant read and write access using temporary access permissions. When you create an Intent
containing a content URI, in order to send the content URI to a client app, you can also call Intent.setFlags()
to add permissions. These permissions are available to the client app for as long as the stack for a receiving Activity
is active. For an Intent
going to a Service
, the permissions are available as long as the Service
is running.
In comparison, to control access to a file:///
Uri
you have to modify the file system permissions of the underlying file. The permissions you provide become available to any app, and remain in effect until you change them. This level of access is fundamentally insecure.
The increased level of file access security offered by a content URI makes FileProvider a key part of Android's security infrastructure.
This overview of FileProvider includes the following topics:
Defining a FileProvider
Since the default functionality of FileProvider includes content URI generation for files, you don't need to define a subclass in code. Instead, you can include a FileProvider in your app by specifying it entirely in XML. To specify the FileProvider component itself, add a <provider>
element to your app manifest. Set the android:name
attribute to android.support.v4.content.FileProvider
. Set the android:authorities
attribute to a URI authority based on a domain you control; for example, if you control the domain mydomain.com
you should use the authority com.mydomain.fileprovider
. Set the android:exported
attribute to false
; the FileProvider does not need to be public. Set the android:grantUriPermissions attribute to true
, to allow you to grant temporary access to files. For example:
If you want to override any of the default behavior of FileProvider methods, extend the FileProvider class and use the fully-qualified class name in the android:name
attribute of the <provider>
element.
Specifying Available Files
A FileProvider can only generate a content URI for files in directories that you specify beforehand. To specify a directory, specify the its storage area and path in XML, using child elements of the<paths>
element. For example, the following paths
element tells FileProvider that you intend to request content URIs for the images/
subdirectory of your private file area. The <paths>
element must contain one or more of the following child elements:
files/
subdirectory of your app's internal storage area. This subdirectory is the same as the value returned by Context.getFilesDir()
. getCacheDir()
. Environment.getExternalStorageDirectory()
. Context#getExternalFilesDir(String) Context.getExternalFilesDir(null)
. Context.getExternalCacheDir()
. Context.getExternalMediaDirs()
. Note: this directory is only available on API 21+ devices.
These child elements all use the same attributes:
name='name'
- A URI path segment. To enforce security, this value hides the name of the subdirectory you're sharing. The subdirectory name for this value is contained in the
path
attribute. path='path'
- The subdirectory you're sharing. While the
name
attribute is a URI path segment, thepath
value is an actual subdirectory name. Notice that the value refers to a subdirectory, not an individual file or files. You can't share a single file by its file name, nor can you specify a subset of files using wildcards.
You must specify a child element of <paths>
for each directory that contains files for which you want content URIs. For example, these XML elements specify two directories:
Put the <paths>
element and its children in an XML file in your project. For example, you can add them to a new file called res/xml/file_paths.xml
. To link this file to the FileProvider, add a <meta-data> element as a child of the <provider>
element that defines the FileProvider. Set the <meta-data>
element's 'android:name' attribute to android.support.FILE_PROVIDER_PATHS
. Set the element's 'android:resource' attribute to @xml/file_paths
(notice that you don't specify the .xml
extension). For example:
Generating the Content URI for a File
To share a file with another app using a content URI, your app has to generate the content URI. To generate the content URI, create a new File
for the file, then pass the File
to getUriForFile()
. You can send the content URI returned by getUriForFile()
to another app in an Intent
. The client app that receives the content URI can open the file and access its contents by calling ContentResolver.openFileDescriptor
to get a ParcelFileDescriptor
.
For example, suppose your app is offering files to other apps with a FileProvider that has the authority com.mydomain.fileprovider
. To get a content URI for the file default_image.jpg
in the images/
subdirectory of your internal storage add the following code: As a result of the previous snippet, getUriForFile()
returns the content URI content://com.mydomain.fileprovider/my_images/default_image.jpg
.
Granting Temporary Permissions to a URI
To grant an access permission to a content URI returned fromgetUriForFile()
, do one of the following: - Call the method
Context.grantUriPermission(package, Uri, mode_flags)
for thecontent://
Uri
, using the desired mode flags. This grants temporary access permission for the content URI to the specified package, according to the value of the themode_flags
parameter, which you can set toFLAG_GRANT_READ_URI_PERMISSION
,FLAG_GRANT_WRITE_URI_PERMISSION
or both. The permission remains in effect until you revoke it by callingrevokeUriPermission()
or until the device reboots. - Put the content URI in an
Intent
by callingsetData()
. - Next, call the method
Intent.setFlags()
with eitherFLAG_GRANT_READ_URI_PERMISSION
orFLAG_GRANT_WRITE_URI_PERMISSION
or both. - Finally, send the
Intent
to another app. Most often, you do this by callingsetResult()
.Permissions granted in an
Intent
remain in effect while the stack of the receivingActivity
is active. When the stack finishes, the permissions are automatically removed. Permissions granted to oneActivity
in a client app are automatically extended to other components of that app.
Serving a Content URI to Another App
There are a variety of ways to serve the content URI for a file to a client app. One common way is for the client app to start your app by calling startActivityResult()
, which sends an Intent
to your app to start an Activity
in your app. In response, your app can immediately return a content URI to the client app or present a user interface that allows the user to pick a file. In the latter case, once the user picks the file your app can return its content URI. In both cases, your app returns the content URI in an Intent
sent via setResult()
.
You can also put the content URI in a ClipData
object and then add the object to an Intent
you send to a client app. To do this, call Intent.setClipData()
. When you use this approach, you can add multiple ClipData
objects to the Intent
, each with its own content URI. When you call Intent.setFlags()
on the Intent
to set temporary access permissions, the same permissions are applied to all of the content URIs.
Note: The Intent.setClipData()
method is only available in platform version 16 (Android 4.1) and later. If you want to maintain compatibility with previous versions, you should send one content URI at a time in the Intent
. Set the action to ACTION_SEND
and put the URI in data by calling setData()
.
More Information
To learn more about FileProvider, see the Android training class Sharing Files Securely with URIs.
Summary
Inherited constants | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
From interface android.content.ComponentCallbacks2
|
Public constructors | |
---|---|
FileProvider() |
Public methods | |
---|---|
void | attachInfo(Context context, ProviderInfo info) After the FileProvider is instantiated, this method is called to provide the system with information about the provider. |
int | delete(Uri uri, String selection, String[] selectionArgs) Deletes the file associated with the specified content URI, as returned by |
String | getType(Uri uri) Returns the MIME type of a content URI returned by |
static Uri | getUriForFile(Context context, String authority, File file) Return a content URI for a given |
Uri | insert(Uri uri, ContentValues values) By default, this method throws an |
boolean | onCreate() The default FileProvider implementation does not need to be initialized. |
ParcelFileDescriptor | openFile(Uri uri, String mode) By default, FileProvider automatically returns the |
Cursor | query(Uri uri, String[] projection, String selection, String[] selectionArgs, String sortOrder) Use a content URI returned by |
int | update(Uri uri, ContentValues values, String selection, String[] selectionArgs) By default, this method throws an |
Inherited methods | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Fromclassandroid.content.ContentProvider
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Fromclass java.lang.Object
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Frominterfaceandroid.content.ComponentCallbacks2
| |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Frominterfaceandroid.content.ComponentCallbacks
|
Public constructors
FileProvider
Public methods
attachInfo
After the FileProvider is instantiated, this method is called to provide the system with information about the provider.
Parameters | |
---|---|
context | Context : A Context for the current component. |
info | ProviderInfo : A ProviderInfo for the new provider. |
delete
Deletes the file associated with the specified content URI, as returned by getUriForFile()
. Notice that this method does not throw an IOException
; you must check its return value.
Parameters | |
---|---|
uri | Uri : A content URI for a file, as returned by getUriForFile() . |
selection | String : Ignored. Set to null . |
selectionArgs | String : Ignored. Set to null . |
Returns | |
---|---|
int | 1 if the delete succeeds; otherwise, 0. |
getType
Returns the MIME type of a content URI returned by getUriForFile()
.
Parameters | |
---|---|
uri | Uri : A content URI returned by getUriForFile() . |
Returns | |
---|---|
String | If the associated file has an extension, the MIME type associated with that extension; otherwise application/octet-stream . |
getUriForFile
Return a content URI for a given File
. Specific temporary permissions for the content URI can be set with grantUriPermission(String, Uri, int)
, or added to an Intent
by calling setData()
and then setFlags()
; in both cases, the applicable flags are FLAG_GRANT_READ_URI_PERMISSION
and FLAG_GRANT_WRITE_URI_PERMISSION
. A FileProvider can only return a content
Uri
for file paths defined in their <paths>
meta-data element. See the Class Overview for more information.
Parameters | |
---|---|
context | Context : A Context for the current component. |
authority | String : The authority of a FileProvider defined in a <provider> element in your app's manifest. |
file | File : A File pointing to the filename for which you want a content Uri . |
Returns | |
---|---|
Uri | A content URI for the file. |
Throws | |
---|---|
IllegalArgumentException | When the given File is outside the paths supported by the provider. |
insert
By default, this method throws an UnsupportedOperationException
. You must subclass FileProvider if you want to provide different functionality.
Parameters | |
---|---|
uri | Uri |
values | ContentValues |
Returns | |
---|---|
Uri |
onCreate
The default FileProvider implementation does not need to be initialized. If you want to override this method, you must provide your own subclass of FileProvider.
Returns | |
---|---|
boolean |
openFile
By default, FileProvider automatically returns the ParcelFileDescriptor
for a file associated with a content://
Uri
. To get the ParcelFileDescriptor
, call ContentResolver.openFileDescriptor
. To override this method, you must provide your own subclass of FileProvider.
Parameters | |
---|---|
uri | Uri : A content URI associated with a file, as returned by getUriForFile() . |
mode | String : Access mode for the file. May be 'r' for read-only access, 'rw' for read and write access, or 'rwt' for read and write access that truncates any existing file. |
Returns | |
---|---|
ParcelFileDescriptor | A new ParcelFileDescriptor with which you can access the file. |
Throws | |
---|---|
FileNotFoundException |
query
Use a content URI returned by getUriForFile()
to get information about a file managed by the FileProvider. FileProvider reports the column names defined in OpenableColumns
:
ContentProvider.query()
.Parameters | |
---|---|
uri | Uri : A content URI returned by getUriForFile(Context, String, File) . |
projection | String : The list of columns to put into the Cursor . If null all columns are included. |
selection | String : Selection criteria to apply. If null then all data that matches the content URI is returned. |
selectionArgs | String : An array of String , containing arguments to bind to the selection parameter. The query method scans selection from left to right and iterates through selectionArgs, replacing the current '?' character in selection with the value at the current position in selectionArgs. The values are bound to selection as String values. |
sortOrder | String : A String containing the column name(s) on which to sort the resulting Cursor . |
Returns | |
---|---|
Cursor | A Cursor containing the results of the query. |
update
By default, this method throws an UnsupportedOperationException
. You must subclass FileProvider if you want to provide different functionality.
Parameters | |
---|---|
uri | Uri |
values | ContentValues |
selection | String |
selectionArgs | String |
Returns | |
---|---|
int |
Warframe Stuck At Verifying Download Cache
Interfaces
Classes
public static final class Manifest.permission
extends Object
java.lang.Object | |
↳ | android.Manifest.permission |
Summary
Constants | |
---|---|
String | ACCEPT_HANDOVER Allows a calling app to continue a call which was started in another app. |
String | ACCESS_BACKGROUND_LOCATION Allows an app to access location in the background. |
String | ACCESS_CHECKIN_PROPERTIES Allows read/write access to the 'properties' table in the checkin database, to change values that get uploaded. |
String | ACCESS_COARSE_LOCATION Allows an app to access approximate location. |
String | ACCESS_FINE_LOCATION Allows an app to access precise location. |
String | ACCESS_LOCATION_EXTRA_COMMANDS Allows an application to access extra location provider commands. |
String | ACCESS_MEDIA_LOCATION Allows an application to access any geographic locations persisted in the user's shared collection. |
String | ACCESS_NETWORK_STATE Allows applications to access information about networks. |
String | ACCESS_NOTIFICATION_POLICY Marker permission for applications that wish to access notification policy. |
String | ACCESS_WIFI_STATE Allows applications to access information about Wi-Fi networks. |
String | ACCOUNT_MANAGER Allows applications to call into AccountAuthenticators. |
String | ACTIVITY_RECOGNITION Allows an application to recognize physical activity. |
String | ADD_VOICEMAIL Allows an application to add voicemails into the system. |
String | ANSWER_PHONE_CALLS Allows the app to answer an incoming phone call. |
String | BATTERY_STATS Allows an application to collect battery statistics Protection level: signature privileged development |
String | BIND_ACCESSIBILITY_SERVICE Must be required by an |
String | BIND_APPWIDGET Allows an application to tell the AppWidget service which application can access AppWidget's data. |
String | BIND_AUTOFILL_SERVICE Must be required by a |
String | BIND_CALL_REDIRECTION_SERVICE Must be required by a |
String | BIND_CARRIER_MESSAGING_CLIENT_SERVICE A subclass of |
String | BIND_CARRIER_MESSAGING_SERVICE This constant was deprecated in API level 23. Use |
String | BIND_CARRIER_SERVICES The system process that is allowed to bind to services in carrier apps will have this permission. |
String | BIND_CHOOSER_TARGET_SERVICE Must be required by a |
String | BIND_CONDITION_PROVIDER_SERVICE Must be required by a |
String | BIND_DEVICE_ADMIN Must be required by device administration receiver, to ensure that only the system can interact with it. |
String | BIND_DREAM_SERVICE Must be required by an |
String | BIND_INCALL_SERVICE Must be required by a |
String | BIND_INPUT_METHOD Must be required by an |
String | BIND_MIDI_DEVICE_SERVICE Must be required by an |
String | BIND_NFC_SERVICE Must be required by a |
String | BIND_NOTIFICATION_LISTENER_SERVICE Must be required by an |
String | BIND_PRINT_SERVICE Must be required by a |
String | BIND_QUICK_SETTINGS_TILE Allows an application to bind to third party quick settings tiles. |
String | BIND_REMOTEVIEWS Must be required by a |
String | BIND_SCREENING_SERVICE Must be required by a |
String | BIND_TELECOM_CONNECTION_SERVICE Must be required by a |
String | BIND_TEXT_SERVICE Must be required by a TextService (e.g. |
String | BIND_TV_INPUT Must be required by a |
String | BIND_VISUAL_VOICEMAIL_SERVICE Must be required by a link |
String | BIND_VOICE_INTERACTION Must be required by a |
String | BIND_VPN_SERVICE Must be required by a |
String | BIND_VR_LISTENER_SERVICE Must be required by an |
String | BIND_WALLPAPER Must be required by a |
String | BLUETOOTH Allows applications to connect to paired bluetooth devices. |
String | BLUETOOTH_ADMIN Allows applications to discover and pair bluetooth devices. |
String | BLUETOOTH_PRIVILEGED Allows applications to pair bluetooth devices without user interaction, and to allow or disallow phonebook access or message access. |
String | BODY_SENSORS Allows an application to access data from sensors that the user uses to measure what is happening inside his/her body, such as heart rate. |
String | BROADCAST_PACKAGE_REMOVED Allows an application to broadcast a notification that an application package has been removed. |
String | BROADCAST_SMS Allows an application to broadcast an SMS receipt notification. |
String | BROADCAST_STICKY Allows an application to broadcast sticky intents. |
String | BROADCAST_WAP_PUSH Allows an application to broadcast a WAP PUSH receipt notification. |
String | CALL_COMPANION_APP Allows an app which implements the |
String | CALL_PHONE Allows an application to initiate a phone call without going through the Dialer user interface for the user to confirm the call. |
String | CALL_PRIVILEGED Allows an application to call any phone number, including emergency numbers, without going through the Dialer user interface for the user to confirm the call being placed. |
String | CAMERA Required to be able to access the camera device. |
String | CAPTURE_AUDIO_OUTPUT Allows an application to capture audio output. |
String | CHANGE_COMPONENT_ENABLED_STATE Allows an application to change whether an application component (other than its own) is enabled or not. |
String | CHANGE_CONFIGURATION Allows an application to modify the current configuration, such as locale. |
String | CHANGE_NETWORK_STATE Allows applications to change network connectivity state. |
String | CHANGE_WIFI_MULTICAST_STATE Allows applications to enter Wi-Fi Multicast mode. |
String | CHANGE_WIFI_STATE Allows applications to change Wi-Fi connectivity state. |
String | CLEAR_APP_CACHE Allows an application to clear the caches of all installed applications on the device. |
String | CONTROL_LOCATION_UPDATES Allows enabling/disabling location update notifications from the radio. |
String | DELETE_CACHE_FILES Old permission for deleting an app's cache files, no longer used, but signals for us to quietly ignore calls instead of throwing an exception. |
String | DELETE_PACKAGES Allows an application to delete packages. |
String | DIAGNOSTIC Allows applications to RW to diagnostic resources. |
String | DISABLE_KEYGUARD Allows applications to disable the keyguard if it is not secure. |
String | DUMP Allows an application to retrieve state dump information from system services. |
String | EXPAND_STATUS_BAR Allows an application to expand or collapse the status bar. |
String | FACTORY_TEST Run as a manufacturer test application, running as the root user. |
String | FOREGROUND_SERVICE Allows a regular application to use |
String | GET_ACCOUNTS Allows access to the list of accounts in the Accounts Service. |
String | GET_ACCOUNTS_PRIVILEGED Allows access to the list of accounts in the Accounts Service. |
String | GET_PACKAGE_SIZE Allows an application to find out the space used by any package. |
String | GET_TASKS This constant was deprecated in API level 21. No longer enforced. |
String | GLOBAL_SEARCH This permission can be used on content providers to allow the global search system to access their data. |
String | INSTALL_LOCATION_PROVIDER Allows an application to install a location provider into the Location Manager. |
String | INSTALL_PACKAGES Allows an application to install packages. |
String | INSTALL_SHORTCUT Allows an application to install a shortcut in Launcher. |
String | INSTANT_APP_FOREGROUND_SERVICE Allows an instant app to create foreground services. |
String | INTERNET Allows applications to open network sockets. |
String | KILL_BACKGROUND_PROCESSES Allows an application to call |
String | LOCATION_HARDWARE Allows an application to use location features in hardware, such as the geofencing api. |
String | MANAGE_DOCUMENTS Allows an application to manage access to documents, usually as part of a document picker. |
String | MANAGE_OWN_CALLS Allows a calling application which manages it own calls through the self-managed |
String | MASTER_CLEAR Not for use by third-party applications. |
String | MEDIA_CONTENT_CONTROL Allows an application to know what content is playing and control its playback. |
String | MODIFY_AUDIO_SETTINGS Allows an application to modify global audio settings. |
String | MODIFY_PHONE_STATE Allows modification of the telephony state - power on, mmi, etc. |
String | MOUNT_FORMAT_FILESYSTEMS Allows formatting file systems for removable storage. |
String | MOUNT_UNMOUNT_FILESYSTEMS Allows mounting and unmounting file systems for removable storage. |
String | NFC Allows applications to perform I/O operations over NFC. |
String | NFC_TRANSACTION_EVENT Allows applications to receive NFC transaction events. |
String | PACKAGE_USAGE_STATS Allows an application to collect component usage statistics Declaring the permission implies intention to use the API and the user of the device can grant permission through the Settings application. |
String | PERSISTENT_ACTIVITY This constant was deprecated in API level 15. This functionality will be removed in the future; please do not use. Allow an application to make its activities persistent. |
String | PROCESS_OUTGOING_CALLS This constant was deprecated in API level 29. Applications should use |
String | READ_CALENDAR Allows an application to read the user's calendar data. |
String | READ_CALL_LOG Allows an application to read the user's call log. |
String | READ_CONTACTS Allows an application to read the user's contacts data. |
String | READ_EXTERNAL_STORAGE Allows an application to read from external storage. |
String | READ_INPUT_STATE This constant was deprecated in API level 16. The API that used this permission has been removed. |
String | READ_LOGS Allows an application to read the low-level system log files. |
String | READ_PHONE_NUMBERS Allows read access to the device's phone number(s). |
String | READ_PHONE_STATE Allows read only access to phone state, including the phone number of the device, current cellular network information, the status of any ongoing calls, and a list of any |
String | READ_SMS Allows an application to read SMS messages. |
String | READ_SYNC_SETTINGS Allows applications to read the sync settings. |
String | READ_SYNC_STATS Allows applications to read the sync stats. |
String | READ_VOICEMAIL Allows an application to read voicemails in the system. |
String | REBOOT Required to be able to reboot the device. |
String | RECEIVE_BOOT_COMPLETED Allows an application to receive the |
String | RECEIVE_MMS Allows an application to monitor incoming MMS messages. |
String | RECEIVE_SMS Allows an application to receive SMS messages. |
String | RECEIVE_WAP_PUSH Allows an application to receive WAP push messages. |
String | RECORD_AUDIO Allows an application to record audio. |
String | REORDER_TASKS Allows an application to change the Z-order of tasks. |
String | REQUEST_COMPANION_RUN_IN_BACKGROUND Allows a companion app to run in the background. |
String | REQUEST_COMPANION_USE_DATA_IN_BACKGROUND Allows a companion app to use data in the background. |
String | REQUEST_DELETE_PACKAGES Allows an application to request deleting packages. |
String | REQUEST_IGNORE_BATTERY_OPTIMIZATIONS Permission an application must hold in order to use |
String | REQUEST_INSTALL_PACKAGES Allows an application to request installing packages. |
String | REQUEST_PASSWORD_COMPLEXITY Allows an application to request the screen lock complexity and prompt users to update the screen lock to a certain complexity level. |
String | RESTART_PACKAGES This constant was deprecated in API level 15. The |
String | SEND_RESPOND_VIA_MESSAGE Allows an application (Phone) to send a request to other applications to handle the respond-via-message action during incoming calls. |
String | SEND_SMS Allows an application to send SMS messages. |
String | SET_ALARM Allows an application to broadcast an Intent to set an alarm for the user. |
String | SET_ALWAYS_FINISH Allows an application to control whether activities are immediately finished when put in the background. |
String | SET_ANIMATION_SCALE Modify the global animation scaling factor. |
String | SET_DEBUG_APP Configure an application for debugging. |
String | SET_PREFERRED_APPLICATIONS This constant was deprecated in API level 15. No longer useful, see |
String | SET_PROCESS_LIMIT Allows an application to set the maximum number of (not needed) application processes that can be running. |
String | SET_TIME Allows applications to set the system time. |
String | SET_TIME_ZONE Allows applications to set the system time zone. |
String | SET_WALLPAPER Allows applications to set the wallpaper. |
String | SET_WALLPAPER_HINTS Allows applications to set the wallpaper hints. |
String | SIGNAL_PERSISTENT_PROCESSES Allow an application to request that a signal be sent to all persistent processes. |
String | SMS_FINANCIAL_TRANSACTIONS Allows financial apps to read filtered sms messages. |
String | START_VIEW_PERMISSION_USAGE Allows the holder to start the permission usage screen for an app. |
String | STATUS_BAR Allows an application to open, close, or disable the status bar and its icons. |
String | SYSTEM_ALERT_WINDOW Allows an app to create windows using the type |
String | TRANSMIT_IR Allows using the device's IR transmitter, if available. |
String | UNINSTALL_SHORTCUT Don't use this permission in your app. |
String | UPDATE_DEVICE_STATS Allows an application to update device statistics. |
String | USE_BIOMETRIC Allows an app to use device supported biometric modalities. |
String | USE_FINGERPRINT This constant was deprecated in API level 28. Applications should request |
String | USE_FULL_SCREEN_INTENT Required for apps targeting |
String | USE_SIP Allows an application to use SIP service. |
String | VIBRATE Allows access to the vibrator. |
String | WAKE_LOCK Allows using PowerManager WakeLocks to keep processor from sleeping or screen from dimming. |
String | WRITE_APN_SETTINGS Allows applications to write the apn settings and read sensitive fields of an existing apn settings like user and password. |
String | WRITE_CALENDAR Allows an application to write the user's calendar data. |
String | WRITE_CALL_LOG Allows an application to write (but not read) the user's call log data. |
String | WRITE_CONTACTS Allows an application to write the user's contacts data. |
String | WRITE_EXTERNAL_STORAGE Allows an application to write to external storage. |
String | WRITE_GSERVICES Allows an application to modify the Google service map. |
String | WRITE_SECURE_SETTINGS Allows an application to read or write the secure system settings. |
String | WRITE_SETTINGS Allows an application to read or write the system settings. |
String | WRITE_SYNC_SETTINGS Allows applications to write the sync settings. |
String | WRITE_VOICEMAIL Allows an application to modify and remove existing voicemails in the system. |
Public constructors | |
---|---|
Manifest.permission() |
Inherited methods | |||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
From class java.lang.Object
|
Constants
ACCEPT_HANDOVER
Allows a calling app to continue a call which was started in another app. An example is a video calling app that wants to continue a voice call on the user's mobile network.
When the handover of a call from one app to another takes place, there are two devices which are involved in the handover; the initiating and receiving devices. The initiating device is where the request to handover the call was started, and the receiving device is where the handover request is confirmed by the other party.
This permission protects access to the TelecomManager.acceptHandover(Uri, int, PhoneAccountHandle)
which the receiving side of the handover uses to accept a handover.
Protection level: dangerous
Constant Value: 'android.permission.ACCEPT_HANDOVER'
ACCESS_BACKGROUND_LOCATION
Allows an app to access location in the background. If you're requesting this permission, you must also request either ACCESS_COARSE_LOCATION
or ACCESS_FINE_LOCATION
. Requesting this permission by itself doesn't give you location access.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.ACCESS_BACKGROUND_LOCATION'
ACCESS_CHECKIN_PROPERTIES
Allows read/write access to the 'properties' table in the checkin database, to change values that get uploaded.
Not for use by third-party applications.
Constant Value: 'android.permission.ACCESS_CHECKIN_PROPERTIES'
ACCESS_COARSE_LOCATION
Allows an app to access approximate location. Alternatively, you might want ACCESS_FINE_LOCATION
.
Protection level: dangerous
Constant Value: 'android.permission.ACCESS_COARSE_LOCATION'
ACCESS_FINE_LOCATION
Allows an app to access precise location. Alternatively, you might want ACCESS_COARSE_LOCATION
.
Protection level: dangerous
Constant Value: 'android.permission.ACCESS_FINE_LOCATION'
ACCESS_LOCATION_EXTRA_COMMANDS
Allows an application to access extra location provider commands.
Protection level: normal
Constant Value: 'android.permission.ACCESS_LOCATION_EXTRA_COMMANDS'
ACCESS_MEDIA_LOCATION
Allows an application to access any geographic locations persisted in the user's shared collection.
Protection level: dangerous
Constant Value: 'android.permission.ACCESS_MEDIA_LOCATION'
ACCESS_NETWORK_STATE
Allows applications to access information about networks.
Protection level: normal
Constant Value: 'android.permission.ACCESS_NETWORK_STATE'
ACCESS_NOTIFICATION_POLICY
Marker permission for applications that wish to access notification policy. This permission is not supported on managed profiles.
Protection level: normal
Constant Value: 'android.permission.ACCESS_NOTIFICATION_POLICY'
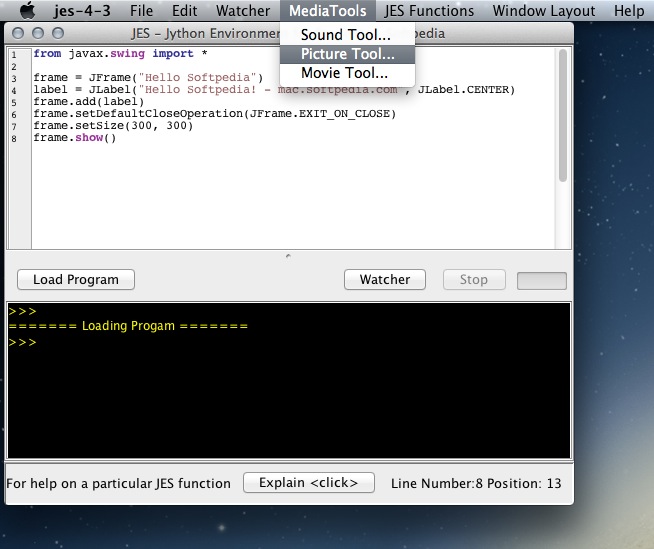
ACCESS_WIFI_STATE
Allows applications to access information about Wi-Fi networks.
Protection level: normal
Constant Value: 'android.permission.ACCESS_WIFI_STATE'
ACCOUNT_MANAGER
Allows applications to call into AccountAuthenticators.
Not for use by third-party applications.
Constant Value: 'android.permission.ACCOUNT_MANAGER'
ACTIVITY_RECOGNITION
Allows an application to recognize physical activity.
Protection level: dangerous
Constant Value: 'android.permission.ACTIVITY_RECOGNITION'
ADD_VOICEMAIL
Allows an application to add voicemails into the system.
Protection level: dangerous
Constant Value: 'com.android.voicemail.permission.ADD_VOICEMAIL'
ANSWER_PHONE_CALLS
Allows the app to answer an incoming phone call.
Protection level: dangerous
Constant Value: 'android.permission.ANSWER_PHONE_CALLS'
BATTERY_STATS
Allows an application to collect battery statistics
Protection level: signature privileged development
Constant Value: 'android.permission.BATTERY_STATS'
BIND_ACCESSIBILITY_SERVICE
Must be required by an AccessibilityService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_ACCESSIBILITY_SERVICE'
BIND_APPWIDGET
Allows an application to tell the AppWidget service which application can access AppWidget's data. The normal user flow is that a user picks an AppWidget to go into a particular host, thereby giving that host application access to the private data from the AppWidget app. An application that has this permission should honor that contract.
Not for use by third-party applications.
Constant Value: 'android.permission.BIND_APPWIDGET'
BIND_AUTOFILL_SERVICE
Must be required by a AutofillService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_AUTOFILL_SERVICE'
BIND_CALL_REDIRECTION_SERVICE
Must be required by a CallRedirectionService
, to ensure that only the system can bind to it.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_CALL_REDIRECTION_SERVICE'
BIND_CARRIER_MESSAGING_CLIENT_SERVICE
A subclass of CarrierMessagingClientService
must be protected with this permission.
Protection level: signature
Constant Value: 'android.permission.BIND_CARRIER_MESSAGING_CLIENT_SERVICE'
BIND_CARRIER_MESSAGING_SERVICE
This constant was deprecated in API level 23.
Use BIND_CARRIER_SERVICES
instead
Constant Value: 'android.permission.BIND_CARRIER_MESSAGING_SERVICE'
BIND_CARRIER_SERVICES
The system process that is allowed to bind to services in carrier apps will have this permission. Carrier apps should use this permission to protect their services that only the system is allowed to bind to.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_CARRIER_SERVICES'
BIND_CHOOSER_TARGET_SERVICE
Must be required by a ChooserTargetService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_CHOOSER_TARGET_SERVICE'
BIND_CONDITION_PROVIDER_SERVICE
Must be required by a ConditionProviderService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_CONDITION_PROVIDER_SERVICE'
BIND_DEVICE_ADMIN
Must be required by device administration receiver, to ensure that only the system can interact with it.
Protection level: signature
Constant Value: 'android.permission.BIND_DEVICE_ADMIN'
BIND_DREAM_SERVICE
Must be required by an DreamService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_DREAM_SERVICE'
BIND_INCALL_SERVICE
Must be required by a InCallService
, to ensure that only the system can bind to it.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_INCALL_SERVICE'
BIND_INPUT_METHOD
Must be required by an InputMethodService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_INPUT_METHOD'
BIND_MIDI_DEVICE_SERVICE
Must be required by an MidiDeviceService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_MIDI_DEVICE_SERVICE'
BIND_NFC_SERVICE
Must be required by a HostApduService
or OffHostApduService
to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_NFC_SERVICE'
BIND_NOTIFICATION_LISTENER_SERVICE
Must be required by an NotificationListenerService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_NOTIFICATION_LISTENER_SERVICE'
BIND_PRINT_SERVICE
Must be required by a PrintService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_PRINT_SERVICE'
BIND_QUICK_SETTINGS_TILE
Allows an application to bind to third party quick settings tiles.
Should only be requested by the System, should be required by TileService declarations.
Constant Value: 'android.permission.BIND_QUICK_SETTINGS_TILE'
BIND_REMOTEVIEWS
Must be required by a RemoteViewsService
, to ensure that only the system can bind to it.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_REMOTEVIEWS'
BIND_SCREENING_SERVICE
Must be required by a CallScreeningService
, to ensure that only the system can bind to it.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_SCREENING_SERVICE'
BIND_TELECOM_CONNECTION_SERVICE
Must be required by a ConnectionService
, to ensure that only the system can bind to it.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_TELECOM_CONNECTION_SERVICE'
BIND_TEXT_SERVICE
Must be required by a TextService (e.g. SpellCheckerService) to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_TEXT_SERVICE'
BIND_TV_INPUT
Must be required by a TvInputService
to ensure that only the system can bind to it.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_TV_INPUT'
BIND_VISUAL_VOICEMAIL_SERVICE
Must be required by a link VisualVoicemailService
to ensure that only the system can bind to it.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_VISUAL_VOICEMAIL_SERVICE'
BIND_VOICE_INTERACTION
Must be required by a VoiceInteractionService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_VOICE_INTERACTION'
BIND_VPN_SERVICE
Must be required by a VpnService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_VPN_SERVICE'
BIND_VR_LISTENER_SERVICE
Must be required by an VrListenerService
, to ensure that only the system can bind to it.
Protection level: signature
Constant Value: 'android.permission.BIND_VR_LISTENER_SERVICE'
BIND_WALLPAPER
Must be required by a WallpaperService
, to ensure that only the system can bind to it.
Protection level: signature privileged
Constant Value: 'android.permission.BIND_WALLPAPER'
BLUETOOTH
Allows applications to connect to paired bluetooth devices.
Protection level: normal
Constant Value: 'android.permission.BLUETOOTH'
BLUETOOTH_ADMIN
Allows applications to discover and pair bluetooth devices.
Protection level: normal
Constant Value: 'android.permission.BLUETOOTH_ADMIN'
BLUETOOTH_PRIVILEGED
Allows applications to pair bluetooth devices without user interaction, and to allow or disallow phonebook access or message access.
Not for use by third-party applications.
Constant Value: 'android.permission.BLUETOOTH_PRIVILEGED'
BODY_SENSORS
Allows an application to access data from sensors that the user uses to measure what is happening inside his/her body, such as heart rate.
Protection level: dangerous
Constant Value: 'android.permission.BODY_SENSORS'
BROADCAST_PACKAGE_REMOVED
Allows an application to broadcast a notification that an application package has been removed.
Not for use by third-party applications.
Constant Value: 'android.permission.BROADCAST_PACKAGE_REMOVED'
BROADCAST_SMS
Allows an application to broadcast an SMS receipt notification.
Not for use by third-party applications.
Constant Value: 'android.permission.BROADCAST_SMS'
BROADCAST_STICKY
Allows an application to broadcast sticky intents. These are broadcasts whose data is held by the system after being finished, so that clients can quickly retrieve that data without having to wait for the next broadcast.
Protection level: normal
Constant Value: 'android.permission.BROADCAST_STICKY'
BROADCAST_WAP_PUSH
Allows an application to broadcast a WAP PUSH receipt notification.
Not for use by third-party applications.
Constant Value: 'android.permission.BROADCAST_WAP_PUSH'
CALL_COMPANION_APP
Allows an app which implements the InCallService
API to be eligible to be enabled as a calling companion app. This means that the Telecom framework will bind to the app's InCallService implementation when there are calls active. The app can use the InCallService API to view information about calls on the system and control these calls.
Protection level: normal
Constant Value: 'android.permission.CALL_COMPANION_APP'
CALL_PHONE
Allows an application to initiate a phone call without going through the Dialer user interface for the user to confirm the call.
Protection level: dangerous
Constant Value: 'android.permission.CALL_PHONE'
CALL_PRIVILEGED
Allows an application to call any phone number, including emergency numbers, without going through the Dialer user interface for the user to confirm the call being placed.
Not for use by third-party applications.
Constant Value: 'android.permission.CALL_PRIVILEGED'
CAMERA
Required to be able to access the camera device.
This will automatically enforce the uses-feature manifest element for all camera features. If you do not require all camera features or can properly operate if a camera is not available, then you must modify your manifest as appropriate in order to install on devices that don't support all camera features.
Protection level: dangerous
Constant Value: 'android.permission.CAMERA'
CAPTURE_AUDIO_OUTPUT
Allows an application to capture audio output. Use the CAPTURE_MEDIA_OUTPUT
permission if only the USAGE_UNKNOWN
), USAGE_MEDIA
) or USAGE_GAME
) usages are intended to be captured.
Not for use by third-party applications.
Constant Value: 'android.permission.CAPTURE_AUDIO_OUTPUT'
CHANGE_COMPONENT_ENABLED_STATE
Allows an application to change whether an application component (other than its own) is enabled or not.
Not for use by third-party applications.
Constant Value: 'android.permission.CHANGE_COMPONENT_ENABLED_STATE'
CHANGE_CONFIGURATION
Allows an application to modify the current configuration, such as locale.
Protection level: signature privileged development
Constant Value: 'android.permission.CHANGE_CONFIGURATION'
CHANGE_NETWORK_STATE
Allows applications to change network connectivity state.
Protection level: normal
Constant Value: 'android.permission.CHANGE_NETWORK_STATE'
CHANGE_WIFI_MULTICAST_STATE
Allows applications to enter Wi-Fi Multicast mode.
Protection level: normal
Constant Value: 'android.permission.CHANGE_WIFI_MULTICAST_STATE'
CHANGE_WIFI_STATE
Allows applications to change Wi-Fi connectivity state.
Protection level: normal
Constant Value: 'android.permission.CHANGE_WIFI_STATE'
CLEAR_APP_CACHE
Allows an application to clear the caches of all installed applications on the device.
Protection level: signature privileged
Constant Value: 'android.permission.CLEAR_APP_CACHE'
CONTROL_LOCATION_UPDATES
Allows enabling/disabling location update notifications from the radio.
Not for use by third-party applications.
Constant Value: 'android.permission.CONTROL_LOCATION_UPDATES'
DELETE_CACHE_FILES
Old permission for deleting an app's cache files, no longer used, but signals for us to quietly ignore calls instead of throwing an exception.
Protection level: signature privileged
Constant Value: 'android.permission.DELETE_CACHE_FILES'
DELETE_PACKAGES
Allows an application to delete packages.
Not for use by third-party applications.
Starting in Build.VERSION_CODES.N
, user confirmation is requested when the application deleting the package is not the same application that installed the package.
Constant Value: 'android.permission.DELETE_PACKAGES'
DIAGNOSTIC
Allows applications to RW to diagnostic resources.
Not for use by third-party applications.
Constant Value: 'android.permission.DIAGNOSTIC'
DISABLE_KEYGUARD
Allows applications to disable the keyguard if it is not secure.
Protection level: normal
Constant Value: 'android.permission.DISABLE_KEYGUARD'
DUMP
Allows an application to retrieve state dump information from system services.
Not for use by third-party applications.
Constant Value: 'android.permission.DUMP'
EXPAND_STATUS_BAR
Allows an application to expand or collapse the status bar.
Protection level: normal
Constant Value: 'android.permission.EXPAND_STATUS_BAR'
FACTORY_TEST
Run as a manufacturer test application, running as the root user. Only available when the device is running in manufacturer test mode.
Not for use by third-party applications.
Constant Value: 'android.permission.FACTORY_TEST'
FOREGROUND_SERVICE
Allows a regular application to use Service.startForeground
.
Protection level: normal
Constant Value: 'android.permission.FOREGROUND_SERVICE'
GET_ACCOUNTS
Allows access to the list of accounts in the Accounts Service.
Note: Beginning with Android 6.0 (API level 23), if an app shares the signature of the authenticator that manages an account, it does not need 'GET_ACCOUNTS'
permission to read information about that account. On Android 5.1 and lower, all apps need 'GET_ACCOUNTS'
permission to read information about any account.
Protection level: dangerous
Constant Value: 'android.permission.GET_ACCOUNTS'
GET_ACCOUNTS_PRIVILEGED
Allows access to the list of accounts in the Accounts Service.
Protection level: signature privileged
Constant Value: 'android.permission.GET_ACCOUNTS_PRIVILEGED'
GET_PACKAGE_SIZE
Allows an application to find out the space used by any package.
Protection level: normal
Constant Value: 'android.permission.GET_PACKAGE_SIZE'
GET_TASKS
This constant was deprecated in API level 21.
No longer enforced.
Constant Value: 'android.permission.GET_TASKS'
GLOBAL_SEARCH
This permission can be used on content providers to allow the global search system to access their data. Typically it used when the provider has some permissions protecting it (which global search would not be expected to hold), and added as a read-only permission to the path in the provider where global search queries are performed. This permission can not be held by regular applications; it is used by applications to protect themselves from everyone else besides global search.
Protection level: signature privileged
Constant Value: 'android.permission.GLOBAL_SEARCH'
INSTALL_LOCATION_PROVIDER
Clear Download Cache
Allows an application to install a location provider into the Location Manager.
Not for use by third-party applications.
Constant Value: 'android.permission.INSTALL_LOCATION_PROVIDER'
INSTALL_PACKAGES
Allows an application to install packages.
Not for use by third-party applications.
Constant Value: 'android.permission.INSTALL_PACKAGES'
INSTALL_SHORTCUT
Allows an application to install a shortcut in Launcher.
In Android O (API level 26) and higher, the INSTALL_SHORTCUT
broadcast no longer has any effect on your app because it's a private, implicit broadcast. Instead, you should create an app shortcut by using the requestPinShortcut()
method from the ShortcutManager
class.
Protection level: normal
3DSMax 2018 + Xforce key, link download google drive, autodesk- 3ds-max– 2018-1-now-includes-3ds- Autodesk 3ds Max 2018. Autodesk® 3ds Max® 2018 software helps deliver improved productivity so that users can work more efficiently and creatively with modelling, animation, rendering and workflow updates. 3ds max 8 xforce keygen download.
Constant Value: 'com.android.launcher.permission.INSTALL_SHORTCUT'
INSTANT_APP_FOREGROUND_SERVICE
Allows an instant app to create foreground services.
Protection level: signature development instant appop
Constant Value: 'android.permission.INSTANT_APP_FOREGROUND_SERVICE'
INTERNET
Allows applications to open network sockets.
Protection level: normal
Constant Value: 'android.permission.INTERNET'
KILL_BACKGROUND_PROCESSES
Allows an application to call ActivityManager.killBackgroundProcesses(String)
.
Protection level: normal
Constant Value: 'android.permission.KILL_BACKGROUND_PROCESSES'
LOCATION_HARDWARE
Allows an application to use location features in hardware, such as the geofencing api.
Not for use by third-party applications.
Constant Value: 'android.permission.LOCATION_HARDWARE'
MANAGE_DOCUMENTS
Allows an application to manage access to documents, usually as part of a document picker.
This permission should only be requested by the platform document management app. This permission cannot be granted to third-party apps.
Constant Value: 'android.permission.MANAGE_DOCUMENTS'
MANAGE_OWN_CALLS
Allows a calling application which manages it own calls through the self-managed ConnectionService
APIs. See PhoneAccount.CAPABILITY_SELF_MANAGED
for more information on the self-managed ConnectionService APIs.
Protection level: normal
Constant Value: 'android.permission.MANAGE_OWN_CALLS'
MASTER_CLEAR
Not for use by third-party applications.
Constant Value: 'android.permission.MASTER_CLEAR'
MEDIA_CONTENT_CONTROL
Allows an application to know what content is playing and control its playback.
Not for use by third-party applications due to privacy of media consumption
Constant Value: 'android.permission.MEDIA_CONTENT_CONTROL'
MODIFY_AUDIO_SETTINGS
Allows an application to modify global audio settings.
Protection level: normal
Constant Value: 'android.permission.MODIFY_AUDIO_SETTINGS'
MODIFY_PHONE_STATE
Allows modification of the telephony state - power on, mmi, etc. Does not include placing calls.
Not for use by third-party applications.
Constant Value: 'android.permission.MODIFY_PHONE_STATE'
MOUNT_FORMAT_FILESYSTEMS
Allows formatting file systems for removable storage.
Not for use by third-party applications.
Constant Value: 'android.permission.MOUNT_FORMAT_FILESYSTEMS'
MOUNT_UNMOUNT_FILESYSTEMS
Allows mounting and unmounting file systems for removable storage.
Not for use by third-party applications.
Constant Value: 'android.permission.MOUNT_UNMOUNT_FILESYSTEMS'
NFC
Allows applications to perform I/O operations over NFC.
Protection level: normal
Constant Value: 'android.permission.NFC'
NFC_TRANSACTION_EVENT
Allows applications to receive NFC transaction events.
Protection level: normal
Constant Value: 'android.permission.NFC_TRANSACTION_EVENT'
PACKAGE_USAGE_STATS
Allows an application to collect component usage statistics
Declaring the permission implies intention to use the API and the user of the device can grant permission through the Settings application.
Protection level: signature privileged development appop
Constant Value: 'android.permission.PACKAGE_USAGE_STATS'
PERSISTENT_ACTIVITY
This constant was deprecated in API level 15.
This functionality will be removed in the future; please do not use. Allow an application to make its activities persistent.
Constant Value: 'android.permission.PERSISTENT_ACTIVITY'
PROCESS_OUTGOING_CALLS
This constant was deprecated in API level 29.
Applications should use CallRedirectionService
instead of the Intent.ACTION_NEW_OUTGOING_CALL
broadcast.
Allows an application to see the number being dialed during an outgoing call with the option to redirect the call to a different number or abort the call altogether.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.PROCESS_OUTGOING_CALLS'
READ_CALENDAR
Allows an application to read the user's calendar data.
Protection level: dangerous
Constant Value: 'android.permission.READ_CALENDAR'
READ_CALL_LOG
Allows an application to read the user's call log.
Note: If your app uses the READ_CONTACTS
permission and both your minSdkVersion
and targetSdkVersion
values are set to 15 or lower, the system implicitly grants your app this permission. If you don't need this permission, be sure your targetSdkVersion
is 16 or higher.
Zettai Karen Children: The Unlimited was actually one of the more surprisingly good series I watched out of the Winter 2013 lineup. One of the nice things about this series is while it does have the Zettai Karen Children name slapped to this series, you don't actually have to watch the original Zettai Karen Children to understand the story. Tags: Watch Zettai Karen Children: The Unlimited - Hyoubu Kyousuke Episode 1 English Sub, Download Zettai Karen Children: The Unlimited - Hyoubu Kyousuke Episode 1 English Sub, Anime Zettai Karen Children: The Unlimited - Hyoubu Kyousuke Episode 1 Streaming Online, Watch Unlimited Psychic Squad Episode 1 English Sub, Download Unlimited Psychic. Zettai karen children the unlimited. The Unlimited – Hyoubu Kyousuke Seorang anak yang bernama Kyosuke Hyobu adalah Bos Penjahat Terkuat, P.A.N.D.R.A. Yang melawan pemerintah dan membuat dirinya di tangkap untuk alasan tertentu, didalam penjara dia bertemu seseorang bernama Hinomiya Andy. Credit: D-AnimeSubs. Anime Lainnya: Zettai Karen Children. Download Zettai Karen Children Batch BD Sub Indo, Download Zettai Karen.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.READ_CALL_LOG'
READ_CONTACTS
Allows an application to read the user's contacts data.
Protection level: dangerous
Constant Value: 'android.permission.READ_CONTACTS'
READ_EXTERNAL_STORAGE
Allows an application to read from external storage.
Any app that declares the WRITE_EXTERNAL_STORAGE
permission is implicitly granted this permission.
This permission is enforced starting in API level 19. Before API level 19, this permission is not enforced and all apps still have access to read from external storage. You can test your app with the permission enforced by enabling Protect USB storage under Developer options in the Settings app on a device running Android 4.1 or higher.
Also starting in API level 19, this permission is not required to read/write files in your application-specific directories returned by Context.getExternalFilesDir(String)
and Context.getExternalCacheDir()
.
Note: If both your minSdkVersion
and targetSdkVersion
values are set to 3 or lower, the system implicitly grants your app this permission. If you don't need this permission, be sure your targetSdkVersion
is 4 or higher.
This is a soft restricted permission which cannot be held by an app it its full form until the installer on record whitelists the permission. Specifically, if the permission is whitelisted the holder app can access external storage and the visual and aural media collections while if the permission is not whitelisted the holder app can only access to the visual and aural medial collections. Also the permission is immutably restricted meaning that the whitelist state can be specified only at install time and cannot change until the app is installed. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Protection level: dangerous
Constant Value: 'android.permission.READ_EXTERNAL_STORAGE'
READ_INPUT_STATE
This constant was deprecated in API level 16.
The API that used this permission has been removed.
Allows an application to retrieve the current state of keys and switches.
Not for use by third-party applications.
Constant Value: 'android.permission.READ_INPUT_STATE'
READ_LOGS
Allows an application to read the low-level system log files.
Not for use by third-party applications, because Log entries can contain the user's private information.
Constant Value: 'android.permission.READ_LOGS'
READ_PHONE_NUMBERS
Android Request Permission
Allows read access to the device's phone number(s). This is a subset of the capabilities granted by READ_PHONE_STATE
but is exposed to instant applications.
Protection level: dangerous
Constant Value: 'android.permission.READ_PHONE_NUMBERS'
READ_PHONE_STATE
Allows read only access to phone state, including the phone number of the device, current cellular network information, the status of any ongoing calls, and a list of any PhoneAccount
s registered on the device.
Note: If both your minSdkVersion
and targetSdkVersion
values are set to 3 or lower, the system implicitly grants your app this permission. If you don't need this permission, be sure your targetSdkVersion
is 4 or higher.
Protection level: dangerous
Constant Value: 'android.permission.READ_PHONE_STATE'
READ_SMS
Allows an application to read SMS messages.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.READ_SMS'
READ_SYNC_SETTINGS
Allows applications to read the sync settings.
Protection level: normal
Constant Value: 'android.permission.READ_SYNC_SETTINGS'
READ_SYNC_STATS
Allows applications to read the sync stats.
Protection level: normal
Constant Value: 'android.permission.READ_SYNC_STATS'
READ_VOICEMAIL
Allows an application to read voicemails in the system.
Protection level: signature privileged
Constant Value: 'com.android.voicemail.permission.READ_VOICEMAIL'
REBOOT
Required to be able to reboot the device.
Not for use by third-party applications.
Constant Value: 'android.permission.REBOOT'
RECEIVE_BOOT_COMPLETED
Allows an application to receive the Intent.ACTION_BOOT_COMPLETED
that is broadcast after the system finishes booting. If you don't request this permission, you will not receive the broadcast at that time. Though holding this permission does not have any security implications, it can have a negative impact on the user experience by increasing the amount of time it takes the system to start and allowing applications to have themselves running without the user being aware of them. As such, you must explicitly declare your use of this facility to make that visible to the user.
Protection level: normal
Constant Value: 'android.permission.RECEIVE_BOOT_COMPLETED'
RECEIVE_MMS
Allows an application to monitor incoming MMS messages.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.RECEIVE_MMS'
RECEIVE_SMS
Allows an application to receive SMS messages.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.RECEIVE_SMS'
RECEIVE_WAP_PUSH
Allows an application to receive WAP push messages.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.RECEIVE_WAP_PUSH'
RECORD_AUDIO
Allows an application to record audio.
Protection level: dangerous
Constant Value: 'android.permission.RECORD_AUDIO'
REORDER_TASKS
Allows an application to change the Z-order of tasks.
Protection level: normal
Constant Value: 'android.permission.REORDER_TASKS'
REQUEST_COMPANION_RUN_IN_BACKGROUND
Allows a companion app to run in the background.
Protection level: normal
Constant Value: 'android.permission.REQUEST_COMPANION_RUN_IN_BACKGROUND'
REQUEST_COMPANION_USE_DATA_IN_BACKGROUND
Allows a companion app to use data in the background.
Protection level: normal
Constant Value: 'android.permission.REQUEST_COMPANION_USE_DATA_IN_BACKGROUND'
REQUEST_DELETE_PACKAGES
Allows an application to request deleting packages. Apps targeting APIs Build.VERSION_CODES.P
or greater must hold this permission in order to use Intent.ACTION_UNINSTALL_PACKAGE
or PackageInstaller.uninstall(VersionedPackage, IntentSender)
.
Protection level: normal
Constant Value: 'android.permission.REQUEST_DELETE_PACKAGES'
REQUEST_IGNORE_BATTERY_OPTIMIZATIONS
Permission an application must hold in order to use Settings.ACTION_REQUEST_IGNORE_BATTERY_OPTIMIZATIONS
.
Protection level: normal
Constant Value: 'android.permission.REQUEST_IGNORE_BATTERY_OPTIMIZATIONS'
REQUEST_INSTALL_PACKAGES
Allows an application to request installing packages. Apps targeting APIs greater than 25 must hold this permission in order to use Intent.ACTION_INSTALL_PACKAGE
.
Protection level: signature
Constant Value: 'android.permission.REQUEST_INSTALL_PACKAGES'
REQUEST_PASSWORD_COMPLEXITY
Allows an application to request the screen lock complexity and prompt users to update the screen lock to a certain complexity level.
Protection level: normal
Constant Value: 'android.permission.REQUEST_PASSWORD_COMPLEXITY'
RESTART_PACKAGES
This constant was deprecated in API level 15.
The ActivityManager.restartPackage(String)
API is no longer supported.
Constant Value: 'android.permission.RESTART_PACKAGES'
SEND_RESPOND_VIA_MESSAGE
Allows an application (Phone) to send a request to other applications to handle the respond-via-message action during incoming calls.
Not for use by third-party applications.
Constant Value: 'android.permission.SEND_RESPOND_VIA_MESSAGE'
SEND_SMS
Allows an application to send SMS messages.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.SEND_SMS'
SET_ALARM
Allows an application to broadcast an Intent to set an alarm for the user.
Protection level: normal
Constant Value: 'com.android.alarm.permission.SET_ALARM'
SET_ALWAYS_FINISH
Allows an application to control whether activities are immediately finished when put in the background.
Not for use by third-party applications.
Constant Value: 'android.permission.SET_ALWAYS_FINISH'
SET_ANIMATION_SCALE
Modify the global animation scaling factor.
Not for use by third-party applications.
Constant Value: 'android.permission.SET_ANIMATION_SCALE'
SET_DEBUG_APP
Configure an application for debugging.
Not for use by third-party applications.
Constant Value: 'android.permission.SET_DEBUG_APP'
SET_PREFERRED_APPLICATIONS
This constant was deprecated in API level 15.
No longer useful, see PackageManager.addPackageToPreferred(String)
for details.
Constant Value: 'android.permission.SET_PREFERRED_APPLICATIONS'
SET_PROCESS_LIMIT
Allows an application to set the maximum number of (not needed) application processes that can be running.
Not for use by third-party applications.
Constant Value: 'android.permission.SET_PROCESS_LIMIT'
SET_TIME
Allows applications to set the system time.
Not for use by third-party applications.
Constant Value: 'android.permission.SET_TIME'
SET_TIME_ZONE
Allows applications to set the system time zone.
Not for use by third-party applications.
Constant Value: 'android.permission.SET_TIME_ZONE'
Download Cache Cleaner For Pc
SET_WALLPAPER
Allows applications to set the wallpaper.
Protection level: normal
Constant Value: 'android.permission.SET_WALLPAPER'
SET_WALLPAPER_HINTS
Allows applications to set the wallpaper hints.
Protection level: normal
Constant Value: 'android.permission.SET_WALLPAPER_HINTS'
SIGNAL_PERSISTENT_PROCESSES
Allow an application to request that a signal be sent to all persistent processes.
Not for use by third-party applications.
Constant Value: 'android.permission.SIGNAL_PERSISTENT_PROCESSES'
SMS_FINANCIAL_TRANSACTIONS
Allows financial apps to read filtered sms messages. Protection level: signature appop
Constant Value: 'android.permission.SMS_FINANCIAL_TRANSACTIONS'
START_VIEW_PERMISSION_USAGE
Allows the holder to start the permission usage screen for an app.
Protection level: signature installer
Constant Value: 'android.permission.START_VIEW_PERMISSION_USAGE'
STATUS_BAR
Allows an application to open, close, or disable the status bar and its icons.
Not for use by third-party applications.
Constant Value: 'android.permission.STATUS_BAR'
SYSTEM_ALERT_WINDOW
Allows an app to create windows using the type WindowManager.LayoutParams.TYPE_APPLICATION_OVERLAY
, shown on top of all other apps. Very few apps should use this permission; these windows are intended for system-level interaction with the user.
Note: If the app targets API level 23 or higher, the app user must explicitly grant this permission to the app through a permission management screen. The app requests the user's approval by sending an intent with action Settings.ACTION_MANAGE_OVERLAY_PERMISSION
. The app can check whether it has this authorization by calling Settings.canDrawOverlays()
.
Protection level: signature preinstalled appop pre23 development
Constant Value: 'android.permission.SYSTEM_ALERT_WINDOW'
TRANSMIT_IR
Allows using the device's IR transmitter, if available.
Protection level: normal
Constant Value: 'android.permission.TRANSMIT_IR'
UNINSTALL_SHORTCUT
Don't use this permission in your app.
This permission is no longer supported.
Constant Value: 'com.android.launcher.permission.UNINSTALL_SHORTCUT'
UPDATE_DEVICE_STATS
Allows an application to update device statistics.
Not for use by third-party applications.
Constant Value: 'android.permission.UPDATE_DEVICE_STATS'
USE_BIOMETRIC
Allows an app to use device supported biometric modalities.
Protection level: normal
Constant Value: 'android.permission.USE_BIOMETRIC'
USE_FINGERPRINT
This constant was deprecated in API level 28.
Applications should request USE_BIOMETRIC
instead
Allows an app to use fingerprint hardware.
Protection level: normal
Constant Value: 'android.permission.USE_FINGERPRINT'
USE_FULL_SCREEN_INTENT
Required for apps targeting Build.VERSION_CODES.Q
that want to use notification full screen intents
.
Protection level: normal
Constant Value: 'android.permission.USE_FULL_SCREEN_INTENT'
USE_SIP
Allows an application to use SIP service.
Protection level: dangerous
Constant Value: 'android.permission.USE_SIP'
VIBRATE
Allows access to the vibrator.
Protection level: normal
Constant Value: 'android.permission.VIBRATE'
WAKE_LOCK
Allows using PowerManager WakeLocks to keep processor from sleeping or screen from dimming.
Protection level: normal
Constant Value: 'android.permission.WAKE_LOCK'
WRITE_APN_SETTINGS
Allows applications to write the apn settings and read sensitive fields of an existing apn settings like user and password.
Not for use by third-party applications.
Constant Value: 'android.permission.WRITE_APN_SETTINGS'
WRITE_CALENDAR
Allows an application to write the user's calendar data.
Protection level: dangerous
Constant Value: 'android.permission.WRITE_CALENDAR'
WRITE_CALL_LOG
Allows an application to write (but not read) the user's call log data.
Note: If your app uses the WRITE_CONTACTS
permission and both your minSdkVersion
and targetSdkVersion
values are set to 15 or lower, the system implicitly grants your app this permission. If you don't need this permission, be sure your targetSdkVersion
is 16 or higher.
Protection level: dangerous
This is a hard restricted permission which cannot be held by an app until the installer on record whitelists the permission. For more details see )'>PackageInstaller.SessionParams.setWhitelistedRestrictedPermissions(Set)
.
Constant Value: 'android.permission.WRITE_CALL_LOG'
WRITE_CONTACTS
Allows an application to write the user's contacts data.
Protection level: dangerous
Constant Value: 'android.permission.WRITE_CONTACTS'
WRITE_EXTERNAL_STORAGE
Allows an application to write to external storage.
Note: If both your minSdkVersion
and targetSdkVersion
values are set to 3 or lower, the system implicitly grants your app this permission. If you don't need this permission, be sure your targetSdkVersion
is 4 or higher.
Starting in API level 19, this permission is not required to read/write files in your application-specific directories returned by Context.getExternalFilesDir(String)
and Context.getExternalCacheDir()
.
If this permission is not whitelisted for an app that targets an API level before Build.VERSION_CODES.Q
this permission cannot be granted to apps.
Protection level: dangerous
Constant Value: 'android.permission.WRITE_EXTERNAL_STORAGE'
WRITE_GSERVICES
Allows an application to modify the Google service map.
Not for use by third-party applications.
Download Cache Size Chart
Constant Value: 'android.permission.WRITE_GSERVICES'
WRITE_SECURE_SETTINGS
Allows an application to read or write the secure system settings.
Not for use by third-party applications.
Constant Value: 'android.permission.WRITE_SECURE_SETTINGS'
WRITE_SETTINGS
Allows an application to read or write the system settings.
Note: If the app targets API level 23 or higher, the app user must explicitly grant this permission to the app through a permission management screen. The app requests the user's approval by sending an intent with action Settings.ACTION_MANAGE_WRITE_SETTINGS
. The app can check whether it has this authorization by calling Settings.System.canWrite()
.
Protection level: signature preinstalled appop pre23
Constant Value: 'android.permission.WRITE_SETTINGS'
WRITE_SYNC_SETTINGS
Allows applications to write the sync settings.
Protection level: normal
Constant Value: 'android.permission.WRITE_SYNC_SETTINGS'
WRITE_VOICEMAIL
Allows an application to modify and remove existing voicemails in the system.
Warframe Optimizing Download Cache
Protection level: signature privileged
Android Get Download Cache Directory Permission
Constant Value: 'com.android.voicemail.permission.WRITE_VOICEMAIL'